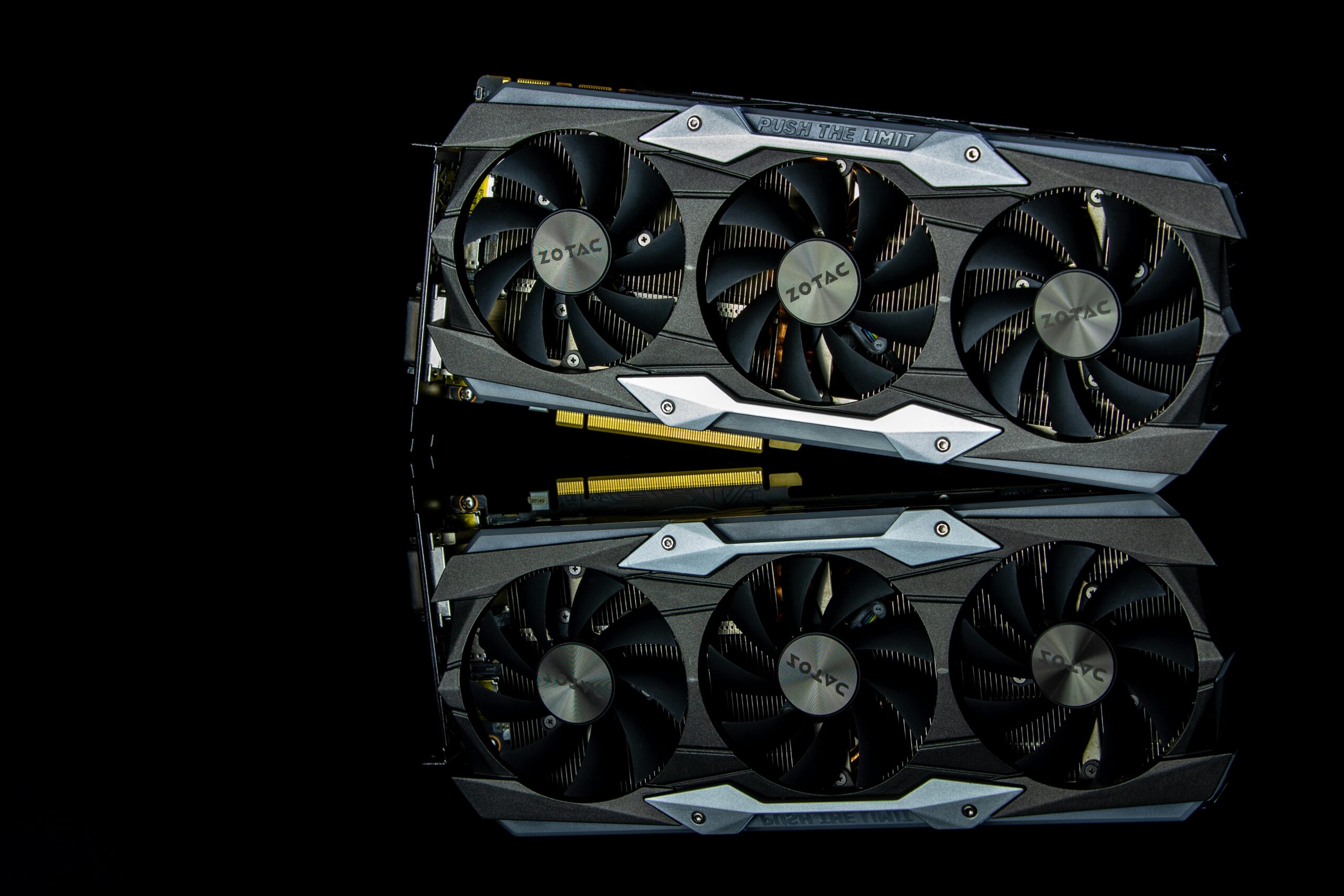
Building a RAG System: End-to-End Process (High-Level)
This document outlines the high-level process of building a Retrieval Augmented Generation (RAG) system, from knowledge base preparation to deployment. We’ll cover each of the different high-level steps and what is required.
1. Knowledge Base Preparation
- Data Collection: Gather your knowledge base documents (text files, PDFs, web pages, etc.).
- Data Cleaning: Clean and pre-process the data (remove irrelevant info, handle special characters, convert to consistent format).
- Chunking: Split the text into smaller, manageable chunks. This is crucial due to LLM context window limitations.
- Storage: Store the chunks in your chosen storage system (S3, R2, Cloud Storage, etc.).
2. Embedding Generation
- Model Selection: Choose an embedding model (e.g., from Gemini, a model on Bedrock, or a third-party embedding API).
- Embedding Creation: Generate vector embeddings for each chunk of text. These embeddings represent the semantic meaning.
- Vector Store: Store the embeddings in a vector database (Pinecone, Weaviate, Vectorize.io, etc.).
3. Application Development (Orchestration)
- User Query: The user submits a query.
- Retrieval: Search the vector database for the most similar embeddings to the query. This retrieves the most relevant context.
- Prompt Construction: Create a prompt for the LLM, including:
- The user’s query.
- The retrieved context chunks.
- Any LLM instructions or formatting.
- LLM Interaction: Send the prompt to the LLM’s API (Gemini API, Grok API, Bedrock API, etc.).
- Response Processing: Process the LLM’s response (extract info, format it, filter it).
- Response Delivery: Send the processed response back to the user.
4. Deployment
- Application Packaging: Package your application code and dependencies (e.g., Docker container, zip file).
- Deployment Platform: Choose a platform (Cloud Functions, Lambda, Workers, Cloud Run, Fargate, etc.).
- Deployment: Deploy your application.
- Testing: Thoroughly test the deployed application.
5. Monitoring and Maintenance
- Monitoring: Track application performance (latency, error rates, user satisfaction).
- Maintenance: Regularly update the knowledge base, embedding model, or application code. Address bugs and issues.
Tools and Technologies
- Programming Languages: Python, JavaScript, or others.
- Frameworks: LangChain (orchestration), Flask/FastAPI (web applications).
- LLMs: Gemini, Grok, models on Bedrock, other LLMs.
- Vector Databases: Pinecone, Weaviate, Chroma, Vectorize.io, etc.
- Cloud Platforms: Google Cloud, AWS, Cloudflare, or others.
- Deployment Platforms: Cloud Functions, Lambda, Workers, Cloud Run, Fargate, etc.
Key Considerations
- Scalability: Design for increasing traffic and data.
- Cost: Optimize resource usage.
- Performance: Minimize latency.
- Security: Secure the application and protect data.
- Maintainability: Write clean, well-documented code.
LangChain and LangFlow
- LangChain: A framework for developing LLM-powered applications. Simplifies orchestration, prompt management, and integration with various services (LLMs, vector stores, etc.). It can be used with *any* of the architectures described above.
- LangFlow: A visual interface for designing LangChain applications, enabling rapid prototyping and collaboration.
Comparing Cloud Providers and Services
Below compares different cloud providers and services for building a Retrieval Augmented Generation (RAG) system, similar to DigitalOcean’s AI Agent, focusing on the key components and their interrelationships.
1. Large Language Model (LLM)
The core model being used for the semantic search.
- DigitalOcean: Offers a choice of LLMs, including open-source models like Llama 2.
- Google: Gemini (through the Gemini API).
- AWS: Amazon Bedrock (for access to foundation models, including Titan, AI21 Labs, Anthropic, Stability AI models) or SageMaker JumpStart (for deploying open-source models).
- xAI: Grok (through the Grok API).
2. Knowledge Base Storage
Where the files are stored that would be used in the search. These are the files that get vectorized.
- DigitalOcean: Spaces (S3-compatible object storage).
- Google:
- Cloud Storage (object storage).
- Firestore (NoSQL document database).
- AWS:
- Amazon S3 (Simple Storage Service – object storage).
- Amazon DynamoDB (NoSQL database).
- Cloudflare: R2 (object storage).
- Other: Any standard file storage system accessible via API (e.g., local file system for testing).
3. Embedding Generation
This is the process of vectorizing the files.
- DigitalOcean: Handled by the platform.
- Google: Gemini API’s embedding endpoint.
- AWS: Amazon Bedrock or SageMaker.
- xAI: Grok API’s embedding endpoint (if available. Check Grok’s API documentation). If Grok doesn’t offer embeddings directly, you would need to use a separate embedding model.
- Cloudflare: Requires an external service (e.g., Gemini API, AWS SageMaker, Grok API if supported, or a third-party API).
- Vectorize.io: Offers managed embedding generation. You point it to your knowledge base storage, and it handles the process using a model of your choice.
4. Vector Database (for Embeddings)
Storage for the vectorized knowledge base
- DigitalOcean: OpenSearch and automatically deployed for the agents. Agents can be assigned to the same one or a different VDB.
- Google:
- Vertex AI Matching Engine (managed).
- OpenSearch (self-managed).
- Third-party vector databases.
- AWS:
- Amazon Kendra (managed search with vector capabilities).
- Amazon OpenSearch Service (managed).
- Third-party vector databases.
- Cloudflare: Requires integration with a third-party vector database (e.g., Pinecone, Weaviate).
- Vectorize.io: Offers a managed vector database as part of its service.
5. Orchestration and Application Logic
- DigitalOcean: Handled by the platform.
- Google: Requires custom development (e.g., using Python/Flask/FastAPI). LangChain can be used to simplify this.
- AWS: Requires custom development (e.g., using Python/Flask/FastAPI). LangChain can be used to simplify this.
- xAI: Requires custom development. LangChain can be used to simplify this.
- Cloudflare: Requires custom development (e.g., using JavaScript with Cloudflare Workers). LangChain can be used to simplify this.
- Vectorize.io: Requires custom development to integrate with their APIs. LangChain can be used to simplify this.
6. Deployment
- DigitalOcean: Handled by the platform.
- Google: Cloud Run, Cloud Functions, App Engine, Compute Engine.
- AWS: AWS Lambda, Fargate, Elastic Beanstalk, EC2.
- xAI: Depends on how you are accessing and using Grok.
- Cloudflare: Cloudflare Workers, or other hosting providers.
- Vectorize.io: You deploy the application that interacts with Vectorize.io.
Example Architectures
DigitalOcean
- Knowledge Base: Spaces (S3-compatible).
- Embedding Generation: Platform handles it.
- Vector Database: OpenSearch.
- Application: Platform handles it.
- Deployment: Platform handles it.
Google Cloud
- Knowledge Base: Cloud Storage.
- Embedding Generation: Gemini API.
- Vector Database: Vertex AI Matching Engine.
- Application: Custom development (e.g., Python/Flask/FastAPI) using LangChain.
- Deployment: Cloud Run, Cloud Functions, etc.
AWS
- Knowledge Base: S3.
- Embedding Generation: Amazon Bedrock or SageMaker.
- Vector Database: Amazon Kendra or Amazon OpenSearch Service.
- Application: Custom development (e.g., Python/Flask/FastAPI) using LangChain.
- Deployment: AWS Lambda, Fargate, etc.
xAI
- Knowledge Base: (e.g., Cloud Storage, S3, R2).
- Embedding Generation: Grok API (if supported) or an external service.
- Vector Database: (e.g., Vertex AI Matching Engine, Amazon Kendra, third-party database).
- Application: Custom development using LangChain.
- Deployment: (e.g., Cloud Run, Lambda, Workers).
Cloudflare
- Knowledge Base: R2.
- Embedding Generation: External service (e.g., Gemini API, AWS SageMaker, Grok API if supported, or a third-party API).
- Vector Database: Third-party vector database.
- Application: Custom development (e.g., JavaScript with Cloudflare Workers) using LangChain.
- Deployment: Cloudflare Workers.
Vectorize.io
- Knowledge Base: (e.g., S3, R2, Cloud Storage). Vectorize.io needs access to this.
- Embedding Generation: Handled by Vectorize.io.
- Vector Database: Managed by Vectorize.io.
- Application: Custom development using LangChain, interacting with Vectorize.io’s API.
- Deployment: (e.g., Cloud Run, Lambda, Workers).
Database Considerations (All Clouds)
- MongoDB: Suitable for complex data relationships, but may be overkill for basic RAG.
- NoSQL Databases (Firestore, DynamoDB): Good for structured storage and complex queries.
- Object Storage (Cloud Storage, S3, Spaces, R2): Simplest and most cost-effective for storing knowledge base files.
Key Differences and Considerations
- Cloudflare: R2’s pricing and CDN integration are attractive. Requires integration with a third-party vector database.
- DigitalOcean: Simplified setup and management.
- Managed vs. Self-Managed (Google/AWS): Consider the trade-offs between ease of use and control.
- Vectorize.io: Simplifies embedding generation and vector database management. Adds a dependency on a third-party service. Consider cost and control trade-offs.